Microsoft Business Applications Blogposts, YouTube Videos and Podcasts
Helping Businesses with Technology
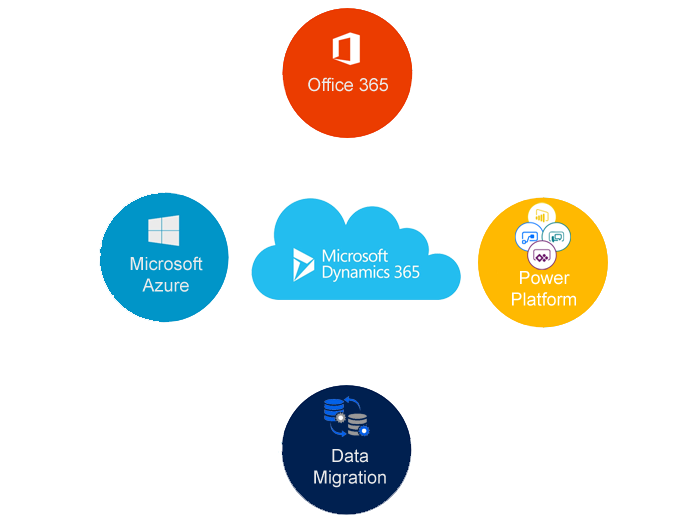
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using ConsoleApplication4;
namespace ConsoleApplication4
{
interface ICustomer1
{
void print1();
}
interface ICustomer2 : ICustomer1
{
void print2();
}
public class Customer : ICustomer2
{
public void print1()
{
Console.WriteLine(“INTERFACE METHOD IMPELMENTED”);
Console.ReadLine();
}
public void print2()
{
Console.WriteLine(“INTERFACE I2METHOD IS IMPLEMENTED TOO”);
Console.ReadLine();
}
}
}
public class Program
{
static void Main()
{
Customer C1 = new Customer();
C1.print1();
C1.print2();
Console.ReadLine();
}
}
===================================================================
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using ConsoleApplication4;
namespace ConsoleApplication4
{
interface I1
{
void INTERFACEMETHOD();
}
interface I2 : I1
{
void INTERFACEMETHOD();
}
public class Program : I1,I2
{
// PUBLIC VOID INTERFACEMETHOD() DEFAULT INTERFACE METHOD
void I1.INTERFACEMETHOD()
{
Console.WriteLine(“INTERFACE I1”);
Console.ReadLine();
}
void I2.INTERFACEMETHOD()
{
Console.WriteLine(“INTERFACE I2”);
Console.ReadLine();
}
static void Main()
{
Program P = new Program();
// P.INTERFACEMETHOD(); DEFAULT INTERFACE CALLING
((I1)P).INTERFACEMETHOD();
((I2)P).INTERFACEMETHOD(); // EXPLICIT INTERFACE METHOD CALLING
Console.ReadLine();
}
}
}
}
}
=====================================================================
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using ConsoleApplication4;
namespace ConsoleApplication4
{
public class Customer
{
public int ID{get; set;}
public string Name{get;set;}
}
}
public class Program
{
static void Main()
{
int i = 0;
if(i==10)
{
int j = 20;
Customer C1 = new Customer();
C1.ID = 101;
C1.Name = “Mark”;
}
Console.WriteLine(“Hello”);
Console.ReadLine();
}
}
=======================
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using ConsoleApplication4;
namespace ConsoleApplication4
{
public class Customer
{
public int ID{get; set;}
public string Name{get;set;}
}
}
public class Program
{
static void Main()
{
int i = 10;
int j = i;
j = j + 1;
Console.WriteLine(“i = {0} && j= {1}”, i, j);
Customer C1 = new Customer();
C1.ID = 101;
C1.Name = “malla”;
// when we copy reference the memory is mapped to same location//
Customer C2 = C1;
C2.Name = “mark”;
Console.WriteLine(“C1.Name = {0}, C2.Name = {1}”, C1.Name, C2.Name);
Console.ReadLine();
}
}
> “classes” can have destructors but “struct” can not have destructors.
Classes vs Structs
> Structs can’t have destructors, but classes can have destructors
> Structs cannot have explicit parameter less constructors where as a class can.
> Struct can’t inherit from another class where as a class can, Both structs and classes can inherit from an interface.
>Example of structs in the .NET Framework -int(System.int32), double(System.Double) etc..
===========================
namespace ConsoleApplication4
{
public class Student
{
private int _Id;
private string _Name;
private int _PassMark = 35;
private string _City;
private string _Email;
public string Email { get; set; }
//get // when there is no logic then we can simply write like above.
//{
// return this._Email;
//}
//set
//{
// this._Email = value;
//}
public string city
{
get
{
return this._City;
}
set
{
this._City = value;
}
}
public int PassMark
{
get
{
return this._PassMark;
}
}
public string Name
{
set{
if (string.IsNullOrEmpty(value))
{
throw new Exception(“NAME SHOULD NOT BE EMPTY OR NULL”);
}
this._Name = value;
}
get
{
return string.IsNullOrEmpty(this._Name) ? “No Name” : this._Name;
}
}
public int Id
{
set
{
if (value <= 0)
{
throw new Exception(“student id is not negative value”);
}
this._Id = value;
}
get
{
return this._Id;
}
}
}
public class Program
{
static void Main()
{
Student C1 = new Student();
C1.Id =101;
C1.Name = “malla”;
Console.WriteLine(“student id = {0}”, C1.Id);
Console.WriteLine(“student Name = {0}”, C1.Name);
Console.WriteLine(“student PassMark = {0}”, C1.PassMark);
Console.ReadLine();
}
}
}
———————————-
namespace ConsoleApplication4
{
public class BaseClass
{
public virtual void print()
{
Console.WriteLine(“I am a Base Class print Method “);
}
}
public class DerivedClass : BaseClass
{
/* public new void print() is for method hiding */
public override void print()
{
Console.WriteLine(“I am a Derived Class print Method “);
}
}
class Program
{
static void Main(string[] args)
{
BaseClass B = new DerivedClass();
B.print();
Console.ReadLine();
}
}
}
======================
METHOD OVERLOADING
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using ConsoleApplication4;
namespace ConsoleApplication4
{
// Method overloading , same method names but different parameters
class Program
{
static void Main(string[] args)
{
Add(2, 3); //N HERE BECAUSE OF STATIC METHOD BELOW WE CAN CALL THE //METHOD WITHOUT INSTANCE
Console.ReadLine();
}
public static void Add(int FN, int LN)
{
Console.WriteLine(“sum = {0} “, FN + LN);
}
public static void Add(int FN, int LN, out int SUM)
{
Console.WriteLine(“I am a Derived Class print Method “);
SUM = FN + LN;
}
}
}